Eto Drawable
Drawable provides infinite flexibility in Eto, you can draw anything in a reusable UI control.
Your first Drawable
Drawables let you draw anything in a 2d space. Use this as a scratch pad and try out the various drawing methods.
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var drawable = new Drawable() { Padding = 5, Width = 200, Height = 200 }; drawable.Paint += (s, e) => { // Circle var rect = new RectangleF(0, 0, 50, 50); e.Graphics.FillEllipse(Colors.Red, rect); // Line var linePen = new Pen(Colors.Blue, 4f); e.Graphics.DrawLine(linePen, 60, 60, 120, 60); // Rectangle var rectPen = new Pen(Colors.Green, 6f); e.Graphics.DrawRectangle(rectPen, 10, 80, 140, 40); // Rounded Rectangle var roundRect = new RectangleF(10, 140, 140, 40); var roundedRectPen = new Pen(Colors.BlueViolet, 6); var roundedRectPath = GraphicsPath.GetRoundRect(roundRect, 10); e.Graphics.DrawPath(roundedRectPen, roundedRectPath); }; var dialog = new Dialog() { Padding = 24, Content = drawable }; dialog.ShowModal(parent);
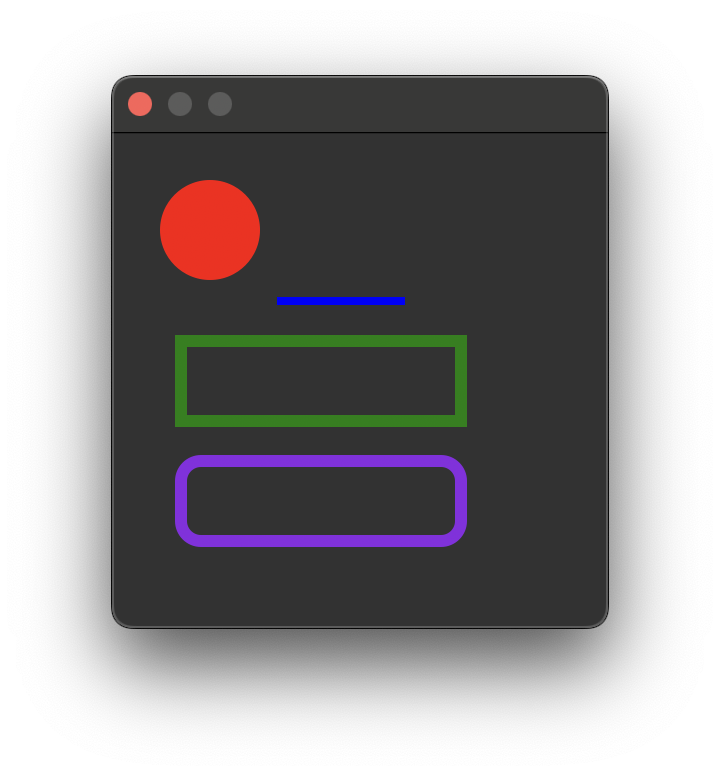
Basic Drawable Mouse Events
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var drawable = new Drawable() { Width = 52, Height = 52 }; bool over; drawable.Paint += (s, e) => { var rect = new RectangleF(1, 1, 50, 50); e.Graphics.FillEllipse(Color.FromArgb(255, 90, 89), rect); if (over) { var pen = new Pen(Color.FromArgb(170, 22, 1), 5f); e.Graphics.DrawLine(pen, 16, 16, 34, 34); e.Graphics.DrawLine(pen, 34, 16, 16, 34); } }; drawable.MouseEnter += (s, e) => { over = true; drawable.Invalidate(true); }; drawable.MouseLeave += (s, e) => { over = false; drawable.Invalidate(true); }; var dialog = new Dialog() { Padding = 24, Content = drawable }; dialog.ShowModal(parent);
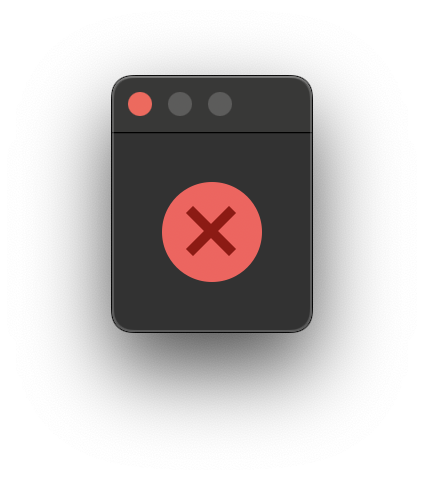
Dragging in Drawables
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); bool mouseDown = false; PointF location = new (100, 100); RectangleF GetRect() { return RectangleF.FromCenter(new PointF(location.X, location.Y), new SizeF(30, 30)); } var drawable = new Drawable() { Width = 200, Height = 200 }; bool over; drawable.Paint += (s, e) => { var rect = GetRect(); e.Graphics.FillEllipse(Colors.OrangeRed, rect); if (!mouseDown) return; e.Graphics.DrawEllipse(Colors.Goldenrod, rect); }; drawable.MouseMove += (s, e) => { if (!mouseDown) return; location = e.Location; drawable.Invalidate(true); }; drawable.MouseUp += (s, e) => { mouseDown = false; drawable.Invalidate(true); }; drawable.MouseDown += (s, e) => { if (!GetRect().Contains(e.Location)) return; mouseDown = true; location = e.Location; drawable.Invalidate(true); }; var dialog = new Dialog() { Padding = 24, Content = drawable }; dialog.ShowInTaskbar = true; dialog.ShowModal();
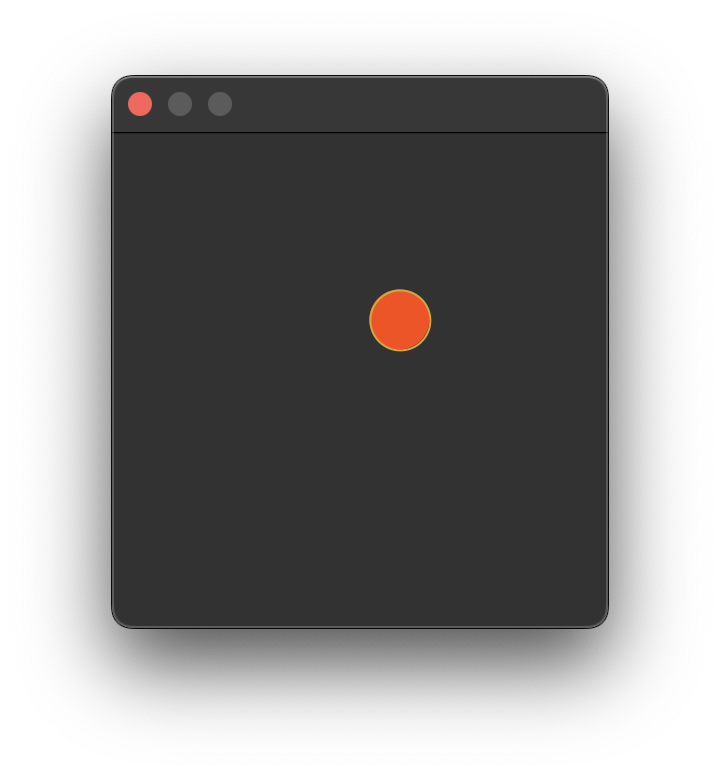