What are Containers?
Containers in eto are controls designed to contain other eto controls. Containers help us create responsive layouts which resize to the dialog or form.
Panels
Panels are a single control container, and mostly used for adding padding.
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var dialog = new Dialog() { Padding = 8, BackgroundColor = Colors.DimGray }; var panel = new Panel() { Padding = 8, BackgroundColor = Colors.DarkGray, Content = new Button() { Text = "Click me!" } }; dialog.Content = panel; dialog.ShowModal(parent);
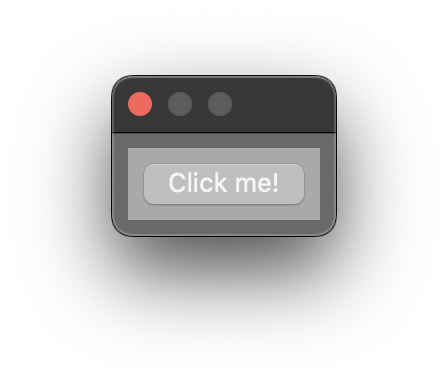
Tables
Table layouts make responsive grids very simple.
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var tableLayout = new TableLayout() { Padding = 8, Spacing = new (4, 4), Rows = { new TableRow(new Button() { Text = "1" }, new Button() { Text = "2" }, new Button() { Text = "3" }), new TableRow(new Button() { Text = "4" }, new Button() { Text = "5" }, new Button() { Text = "6" }), new TableRow(new Button() { Text = "7" }, new Button() { Text = "8" }, new Button() { Text = "9" }), new TableRow(null, new Button() { Text = "0" }, null), } }; var dialog = new Dialog(); dialog.Content = tableLayout; dialog.ShowModal(parent);
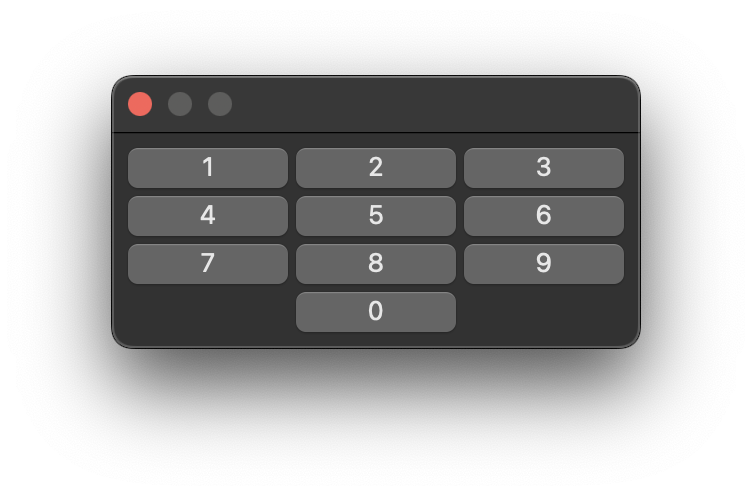
Stack Layouts
Stack Layouts stack a list of controls in a vertical or horizontal direction.
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var dialog = new Dialog(); var stackLayout = new StackLayout() { Spacing = 8, Padding = 8 }; stackLayout.Orientation = Orientation.Vertical; stackLayout.Items.Add(new Button() { Text = "One" }); stackLayout.Items.Add(new Button() { Text = "Two" }); stackLayout.Items.Add(new Button() { Text = "Three" }); dialog.Content = stackLayout; dialog.ShowModal(parent);
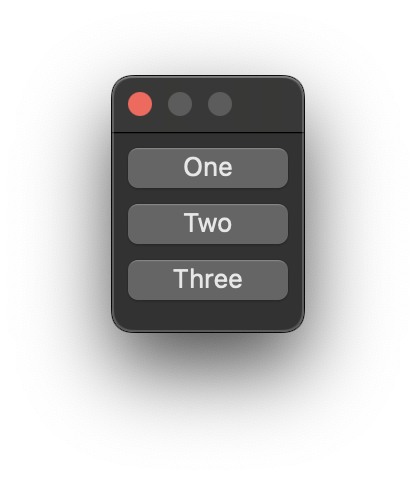
Dynamic Layouts
Dynamic Layouts allow powerful vertical and horizontal organisation of a UI with one (or more) item(s) in the layout stretching to fill the available space.
using Eto.Forms; using Eto.Drawing; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var dialog = new Dialog() { Width = 300, Height = 300 }; var dynamicLayout = new DynamicLayout() { Padding = 8 }; dynamicLayout.BeginVertical(); dynamicLayout.AddSeparateRow(4, null, true, false, new [] { null, new Button() { Text = "Copy", Width = 50 } }); dynamicLayout.Add(new TextArea(), true, true); dynamicLayout.AddSeparateRow(12, new (8, 4), true, false, new [] { new Button() { Text = "Help" }, new Button() { Text = "Cancel" }, new Button() { Text = "Apply" }, }); dynamicLayout.EndVertical(); dialog.Content = dynamicLayout; dialog.ShowModal(parent);
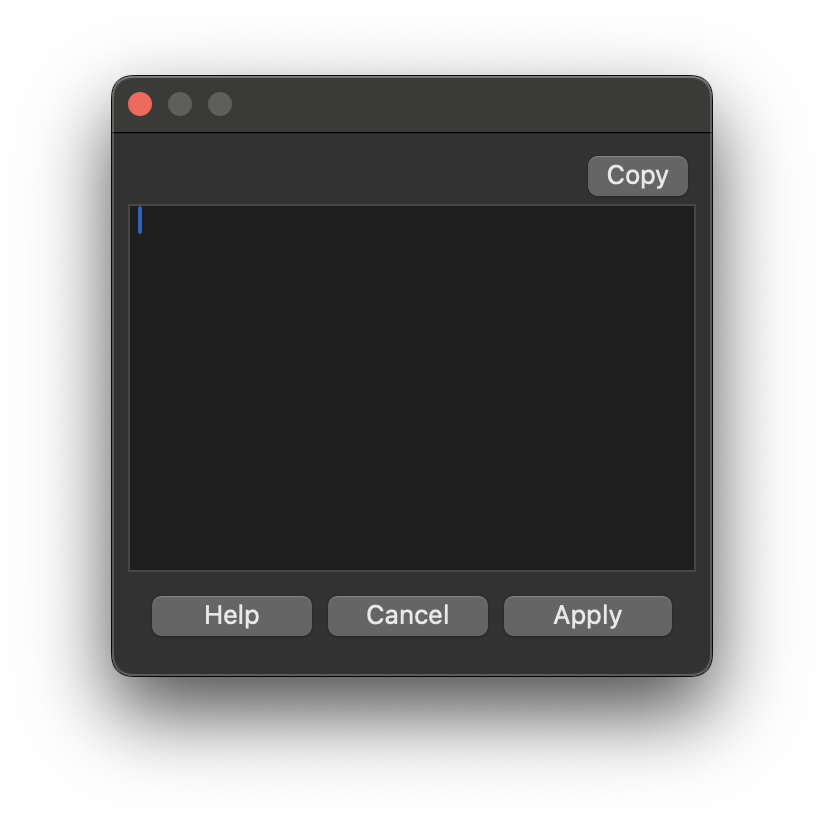
Pixel Layouts
Pixel Layouts allow adding elements at specific pixel coordinates. Pixel Layouts are not a good way to create responsive layouts, any of the above containers should be used instead.
The best use of Pixel Layouts is to overlay elements on top of another, which other layouts do not allow for.
using Eto.Forms; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var dialog = new Dialog() { Padding = 20 }; var pixelLayout = new PixelLayout(); var button1 = new Button() { Text = "Button 1" }; var button2 = new Button() { Text = "Button 2" }; pixelLayout.Add(button1, 0, 0); pixelLayout.Add(button2, 0, 30); dialog.Content = pixelLayout; dialog.ShowModal(parent);
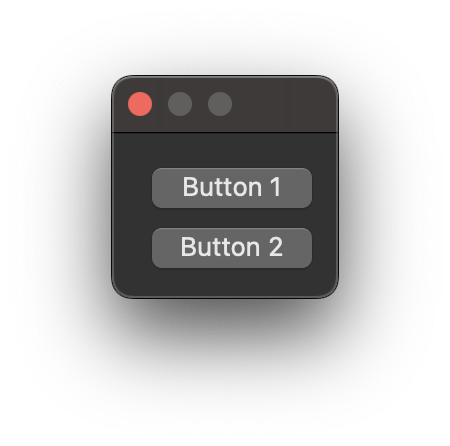