Enabled
Controls are by default enabled, but can be disabled with Enabled = false
. When a control is disabled it will not respond to any of its events, such as mouse interaction or keys,.
using Eto.Forms; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var dialog = new Dialog() { Padding = 8, Content = new Button() { Text = "Disabled", Enabled = false } }; dialog.ShowModal(parent);
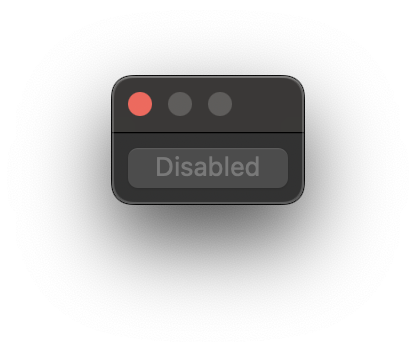
Visible
Controls are by default visible, but can be made invisible with Visible = false
. When a control is invisible it still exists. It will not receive any events such as mouse of keyboard, and may not be considered when the control layout is calculated. Note that the dialog defaults to different size than when the button is visible.
using Eto.Forms; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var dialog = new Dialog() { Width = 200, Height = 200, Padding = 8, Content = new Button() { Text = "Invisible", Visible = false } }; dialog.ShowModal(parent);
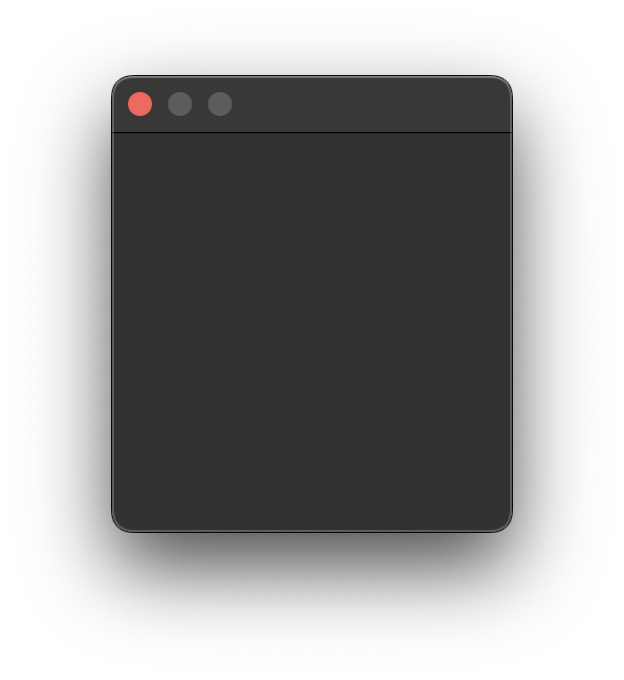
Tag
Tag allows for attaching an arbitrary piece of data to a Control, it is best to keep this piece of data simple. A number, string, enum or struct. Avoid larger items such as images, and especially other controls.
This can be very useful when controls are not hard coded, and instead created in loops.
Parent(s) & ParentWindow
All Controls shown in the UI will have a Parent and ParentWindow. ParentWindow retrieves the Dialog or Form this control is hosted in. This can be very useful when wanting to set focus to the parent or show another window on top of the current window.
Cursor
The mouse cursor can easily be changed per control. This is a very powerful and intuitive way to indicate to the user how the control should be used. For Example indicating Drag functionally by using the grabbing hand cursor.
using Eto.Forms; using Rhino.UI; var parent = RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var button = new Button() { Text = "Move", Cursor = Cursors.Move }; var dialog = new Dialog() { Padding = 28, Content = button, Cursor = Cursors.Crosshair }; dialog.ShowModal(parent);
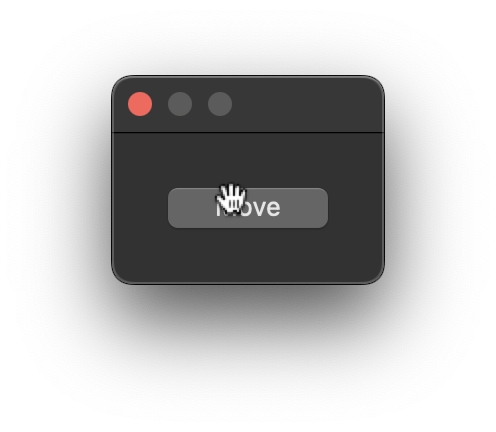