Existing Dialogs
Message Box
MessageBox is a very simple and highly useful dialog for handling control flow and allowing users to make informed decisions.
using Eto.Forms; var result = MessageBox.Show("Would you like to save before closing?", MessageBoxButtons.YesNo, MessageBoxType.Question, MessageBoxDefaultButton.Yes); if (result != DialogResult.Yes) { MessageBox.Show("Model discarded successfully!", MessageBoxButtons.OK, MessageBoxType.Information); } else { MessageBox.Show("Model saved successfully before closing.", MessageBoxButtons.OK, MessageBoxType.Information); }
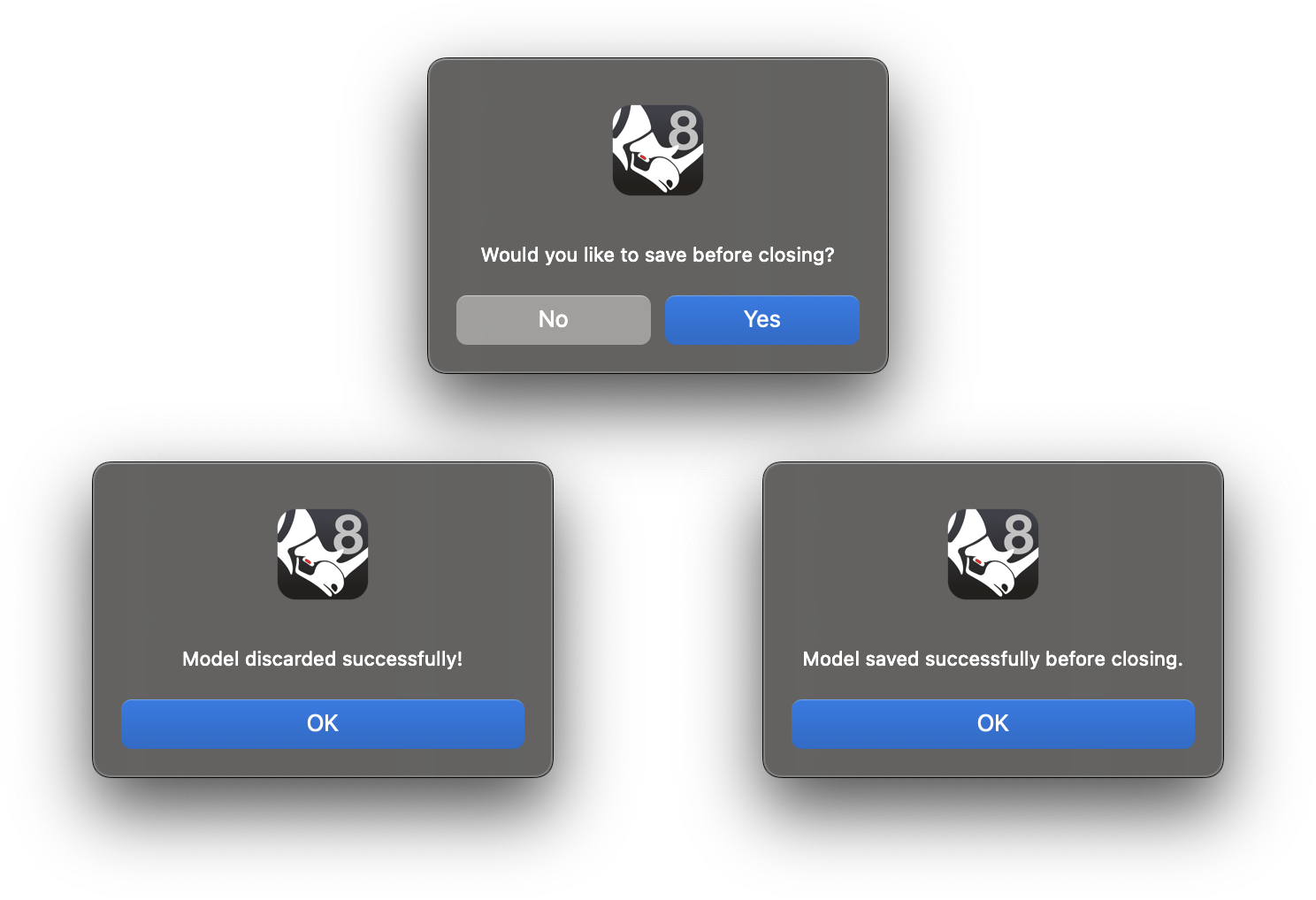
Color Picker
The Color Picker is a button that shows the ColorDialog. The Color Dialog can also be used separately.
using Eto.Forms; var dialog = new Dialog() { Width = 80, Height = 80, Padding = 8, Content = new ColorPicker() }; dialog.ShowModal();
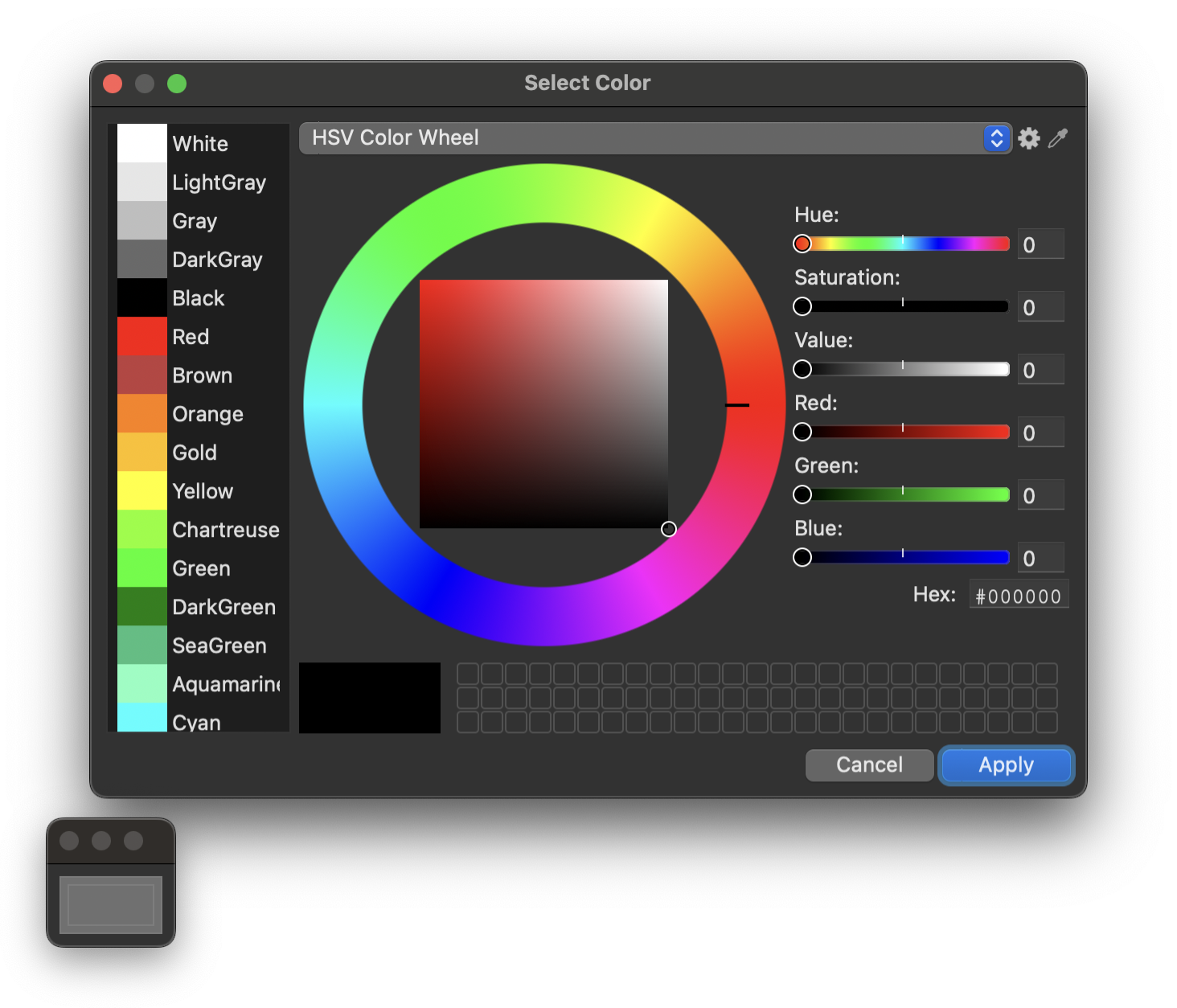
File Dialogs
File dialogs make prompting users to choose new and existing files simple.
If you need to prompt for a folder, there is the SelectFolderDialog
Your Data is Safe
The below example does not save or open any files you choose.
It only shows dialogs that assist in getting file names.
using Eto.Forms; var parent = Rhino.UI.RhinoEtoApp.MainWindowForDocument(__rhino_doc__); var openDialog = new OpenFileDialog() { Filters = { new FileFilter("Any", "*.*") }, CurrentFilterIndex = 0, CheckFileExists = true, MultiSelect = true, Title = "Pick a file, any file" }; var result = openDialog.ShowDialog(parent); if (result == DialogResult.Cancel) { MessageBox.Show("No File chosen!", MessageBoxType.Information); return; } var saveDialog = new SaveFileDialog() { Title = "Save your file", Directory = openDialog.Directory, FileName = openDialog.FileName }; result = saveDialog.ShowDialog(parent); if (result == DialogResult.Cancel) { MessageBox.Show("File not saved!", MessageBoxType.Information); return; }
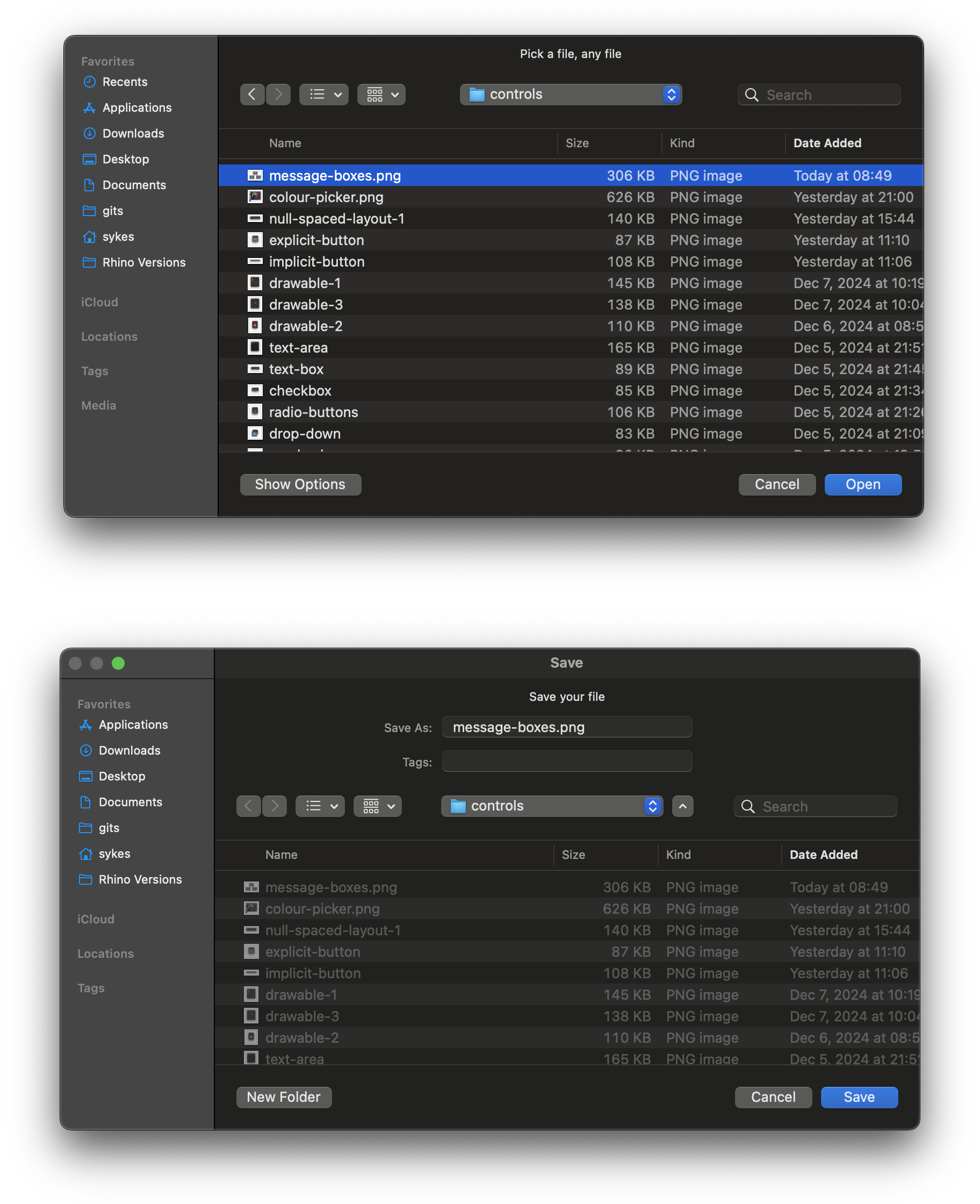